Implementing HTTP Early Hints with Cloudflare Workers ๐
HTTP Early Hints (status code 103) is a powerful feature that allows servers to send hints about critical resources before the main response is ready. This can significantly improve loading performance by letting browsers preload key assets earlier. In this article, we'll implement Early Hints using Cloudflare Workers and R2 storage for a static site.
What are HTTP Early Hints?
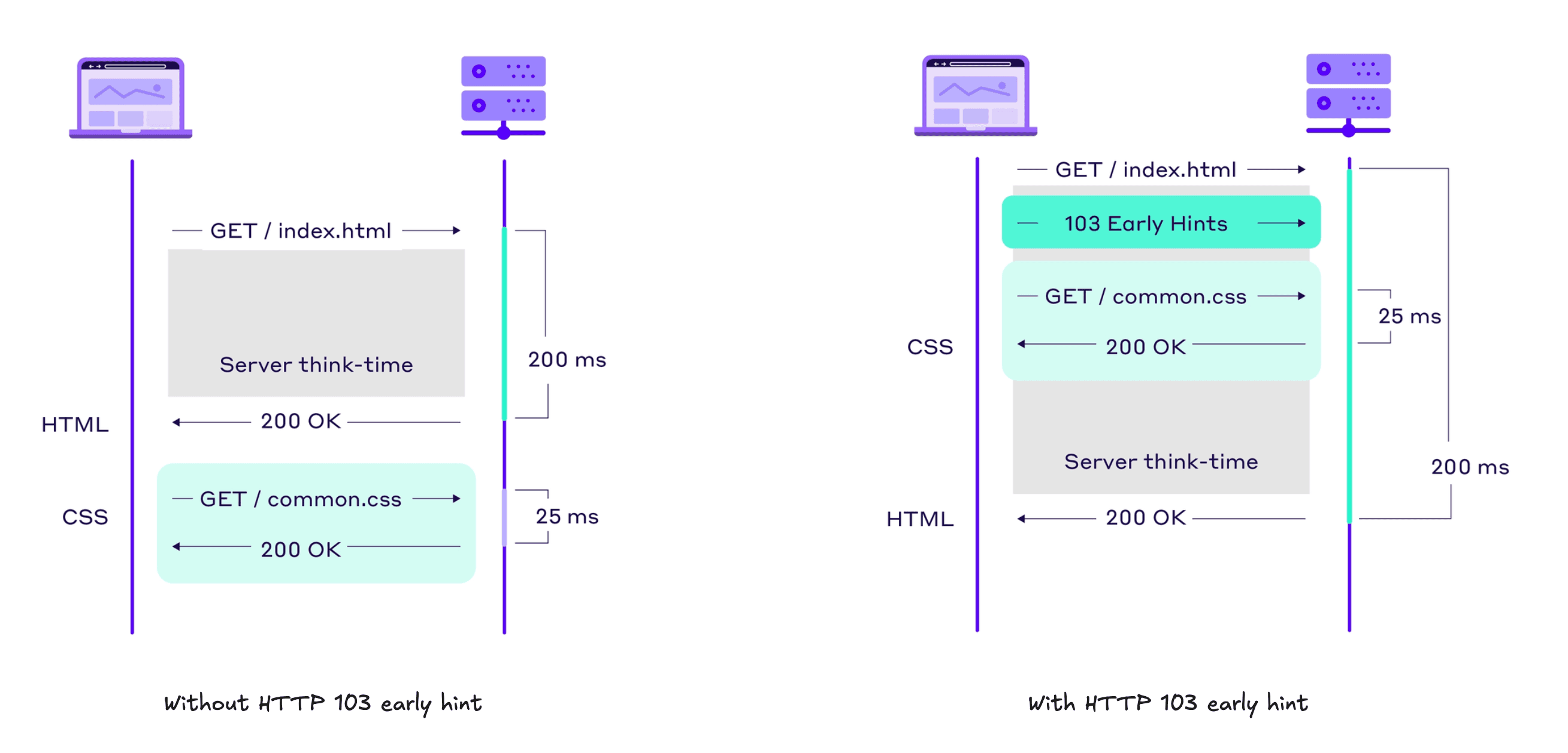
Early Hints is an HTTP status code (103) that enables servers to send preliminary HTTP headers to browsers before the final response is ready. This is particularly useful for indicating resources that the browser will need soon, allowing it to begin loading them earlier in the page lifecycle.
The main benefits include:
- Earlier discovery of critical resources
- Improved perceived loading performance
- Better resource prioritization
Implementation Overview
We'll build a Cloudflare Worker that:
- Serves static files from R2 storage
- Analyzes HTML content to find critical assets
- Adds Early Hints headers for preloading/preconnecting to resources
- Handles proper content encoding and ETags
Let's break down the implementation step by step.
The Worker Implementation
1. Basic Request Handling
First, we set up the basic structure to handle GET and HEAD requests:
export default {
async fetch(request, env, ctx) {
switch (request.method) {
case 'HEAD':
case 'GET':
// Handle static file serving
break;
default:
return new Response('Method Not Allowed', {
status: 405,
headers: {
Allow: 'GET, HEAD',
},
});
}
}
};
2. Path Resolution
We need to handle paths properly, including automatic index.html resolution:
const url = new URL(request.url);
const key = decodeURIComponent(url.pathname);
// Handle trailing slashes and index.html
let objKey = key.replace(/\/+$/gm, '');
if (!/[^\/]+\.[^\/.]+$/.test(key)) {
objKey += '/index.html';
}
3. Serving Files from R2
We fetch the file from R2 storage and handle basic responses:
const object = await env.MY_BUCKET.get(objKey);
if (object === null) {
return new Response('404 Not Found', { status: 404 });
}
const headers = new Headers();
object.writeHttpMetadata(headers);
headers.set('etag', object.httpEtag);
headers.set('Content-Encoding', 'gzip');
4. Early Hints Implementation
The magic happens when serving HTML files. We analyze the content to find resources that should be preloaded:
if (objKey.endsWith('.html')) {
const text = await object.text();
const links = extractEarlyHintsAssets(host, text);
for (const l of links) {
let v = `<${l.url}>; rel=${l.isPreload ? 'preload' : 'preconnect'}`;
if (l.isPreload) v = `${v} as=${l.as}`;
headers.append('link', v);
}
return new Response(text, { headers });
}
5. Asset Detection Logic
We implement sophisticated asset detection that finds critical resources in HTML:
const extractEarlyHintsAssets = (host, body) => {
// Match URLs in link, script, and img tags
const reg = new RegExp(`^(?:/|https?://${host.replaceAll('.', '\\.')}).*$`);
const s = new Set();
return [...body.matchAll(
/<link.+href="([^"]+)".*>|<script.+src="([^"]+)".*>|<img.+src="([^"]+)".*>/gm
)]
.flatMap(match => match.slice(1))
.map(url => {
if (!url) return { url: '' };
// Clean up URL and determine asset type
url = url.split('?')[0];
let as = '';
if (url.endsWith('.css')) as = 'style';
else if (url.endsWith('.js')) as = 'script';
const isPreload = as !== '' && reg.test(url);
// Handle external vs internal resources
if (!isPreload) {
try {
const u = new URL(url);
url = `${u.protocol}//${u.host}`;
} catch {
url = '';
}
} else {
url = url.replace(`https://${host}`, '');
}
return { isPreload, url, as };
})
.filter(link => {
// Deduplicate links
let found = true;
if (s.has(link.url)) found = false;
else s.add(link.url);
return link.url && link.url !== `https://${host}` && found;
});
};
You can find the full code here.
How It Works
- When a request comes in for an HTML file, the worker fetches it from R2
- The HTML content is analyzed to find all resource links (CSS, JS, images)
- For each resource:
- Internal resources (same domain) are marked for preloading
- External resources are marked for preconnect
- Link headers are added to the response for each resource
- The browser can begin loading these resources earlier
Performance Benefits
The Early Hints implementation provides several performance benefits:
- Earlier Resource Discovery: Browsers can discover critical resources before parsing the HTML
- Parallel Loading: Resources can be loaded in parallel with HTML parsing
- Optimized Connection Setup: Preconnect hints allow browsers to set up connections early
- Reduced Time to Interactive: Earlier resource loading can lead to faster page interactivity
Setting Up in Cloudflare
To use this worker:
- Create an R2 bucket in Cloudflare
- Upload your static site files to the bucket
- Deploy the worker code
- Bind the R2 bucket to your worker as
MY_BUCKET
- Set up a route pattern to direct traffic to your worker
Conclusion
HTTP Early Hints is a powerful feature that can significantly improve loading performance for static sites. By implementing it with Cloudflare Workers and R2, we get an efficient and scalable solution that automatically optimizes resource loading for better user experience.
The implementation we've covered automatically detects and hints at critical resources, handles both internal and external resources appropriately, and maintains proper caching headers - all while serving content from Cloudflare's edge network.
Remember that Early Hints is still a relatively new feature, so browser support may vary. However, implementing it now provides a progressive enhancement that will benefit more users as browser support grows.